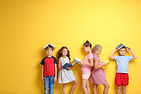

Embed multi attach functionality on pega external assignment for repeating dynamic layout
4
232
0
As Pega external assignment (DWA) doesn't allow AJAX calls due to security concern embedding a repeat list using page list or page group is impossible. In this case if you want to embed a functionality to allow users to attach multiple documents for each of a row in a repeating dynamic layout, follow below solution.
For this solution HTML and Java Script combination should be used to code the solution as the functionality should happen on the client browser side without having AJAX calls.
Create a rule called MultiFileAttach.js (example).
Embed below HTML and Java Script combination code.
In the below java script (Findings is the page list where we want to embed multi attach functionality for each finding). Attachments is the clipboard pagelist name to hold the attachment information.
// Get all table elements with class "bodyTbl_right"
var tables = document.querySelectorAll("[id='bodyTbl_right']");
// Loop through each table element
for (var x = 0; x < tables.length; x++) {
var mytable = tables[x];
var propValue = mytable.getAttribute('pl_prop');
//Delete only the table rows matching with Attachments property
if(propValue==='.Attachments'){
// Delete the rows added to the clipboard from DOM
var rowlength = mytable.rows.length;
var tableHeaderRowCount = 1;
for (var i = tableHeaderRowCount; i < rowlength; i++) {
mytable.deleteRow(tableHeaderRowCount);
}
}
}
function delrow(r) {
var tablenum = r.split('-')[1];
tablenum = (Number(tablenum) * 2) - 1;
var rowlen = r.split('-')[2];
var deltable = tables[tablenum];
// delete selected row
deltable.deleteRow(rowlen);
}
function addrow(num) {
var currtabindex;
currtabindex = (Number(num) * 2) - 1;
var table = tables[currtabindex];
var ref = table.getAttribute("grid_ref_page").charAt(20);
var length = table.rows.length;
var row = table.insertRow(-1);
row.setAttribute("name","$PpyWorkPage$pFindings$l"+ref+"$pAttachments$l"+length);
row.setAttribute("class","cellCont notFocused");
row.setAttribute("style","height:48px;");
var input = document.createElement("input");
input.setAttribute("id", "files-"+ ref +"-"+length);
input.setAttribute("type", "file");
input.setAttribute("name", "fileName");
input.setAttribute("style", "width: 500px");
input.setAttribute("onchange","handleFileSelect(event,this.id)");
var input1 = document.createElement("input");
input1.setAttribute("id", "fileName-"+ ref +"-"+length);
input1.setAttribute("type", "hidden");
input1.setAttribute("name", "$PpyWorkPage$pFindings$l"+ref+"$pAttachments$l"+length+"$ppyFileName");
var input2 = document.createElement("input");
input2.setAttribute("id", "filePath-"+ ref +"-"+length);
input2.setAttribute("type", "hidden");
input2.setAttribute("name", "$PpyWorkPage$pFindings$l"+ref+"$pAttachments$l"+length+"$ppyFilePath");
var input3 = document.createElement("input");
input3.setAttribute("id", "fileContent-"+ ref +"-"+length);
input3.setAttribute("type", "hidden");
input3.setAttribute("name", "$PpyWorkPage$pFindings$l"+ref+"$pAttachments$l"+length+"$ppyNote");
var td = document.createElement("td");
td.setAttribute("data-attribute-name","Attach");
var td1 = document.createElement("td");
td1.setAttribute("data-attribute-name","File Name");
td1.setAttribute("name","BASE_REF");
td1.setAttribute("class","gridCell");
var td2 = document.createElement("td");
td2.setAttribute("data-attribute-name","File Path");
td2.setAttribute("name","BASE_REF");
td2.setAttribute("class","gridCell");
var td3 = document.createElement("td");
td3.setAttribute("data-attribute-name","File Content");
td3.setAttribute("name","BASE_REF");
td3.setAttribute("class","gridCell");
var td4 = document.createElement("td");
var btn = document.createElement("button");
btn.innerHTML = "Delete";
btn.setAttribute("id", "btn-"+ ref +"-"+length);
btn.setAttribute("data-ctl", "Button");
btn.setAttribute("onclick", "delrow(this.id);");
td.appendChild(input);
td1.appendChild(input1);
td2.appendChild(input2);
td3.appendChild(input3);
td4.appendChild(btn);
row.appendChild(td);
row.appendChild(td1);
row.appendChild(td2);
row.appendChild(td3);
row.appendChild(td4);
}
function handleFileSelect(event,id) {
var files = event.target.files;
if (files.length > 0) {
getBase(files[0],id);
}
}
function getBase(file,id) {
var reader = new FileReader();
reader.readAsDataURL(file);
reader.onload = function(){
var refnum = id.split('-')[1];
var rowlength = id.split('-')[2];
var suffix = refnum + "-" +rowlength;
document.getElementById('filePath-'+suffix).value=document.getElementById(id).value;
document.getElementById('fileName-'+suffix).value= document.getElementById(id).value.split("\\").pop();
document.getElementById('fileContent-'+suffix).value= reader.result;
var filePath = document.getElementById(id).value;
pega.api.ui.actions.setValue(("$PpyWorkPage$pFindings$l"+refnum+"$pAttachments$l"+rowlength+"$ppyFileName"), filePath , true, "");
var fileName = document.getElementById(id).value.split("\\").pop();
pega.api.ui.actions.setValue(("$PpyWorkPage$pFindings$l"+refnum+"$pAttachments$l"+rowlength+"$ppyFilePath"), fileName , true, "");
var fileContent = reader.result;
pega.api.ui.actions.setValue(("$PpyWorkPage$pFindings$l"+refnum+"$pAttachmentList$l"+rowlength+"$ppyNote"), fileContent ,true, "");
};
reader.onerror = function (error) {
alert('Error: ', error);
};
}
//end of the script
//static-content-hash-trigger-GCC
Save the file and add MultiFileAttach.js file under Scripts & Styles tab of the PerformExternal harness rule. If PerformExternal is not in your application class save as OOTB harness rule into your application work class to make sure it doesn't break other applications code.
Later go to the internal section which is referred inside the repeating dynamic layout (Data) class section and a Button and Table with Attachments page list.
Refer pyFileName, pyFilePath and any property to show on uploading the file. Add file button adds a row and show Upload File button from Java Script file.
Here is one more twist, to hold the attachments data on the clipabord for post processing, we should instantiate the Attachments page list before loading the external assignment in the pre data transform. For example in this screen we are limiting to 30 documents, so we should create Attachments pagelist with 30 list items but to hide the created page list items on the section we wrote code in the above snippet which makes our screen clean.
That's it now user should be able to upload attachments up to 30 for each finding. And once the documents are uploaded in the post activity we are good to process the data available on the clipboard to link and save the attachments to the case.
Output:
Below screen represents how the upload documents section renders on external assignment.
On click of Add File button.
Before upload:
After upload:
I know it is long post and bored you but you are good to implement the functionality with the above clear representation. Please leave comment and like if it helps.
Related Posts
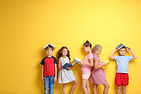